Arithmetic¶
Many programs perform arithmetic calculations. The following table summarizes the arithmetic operators, which include some symbols not used in algebra.
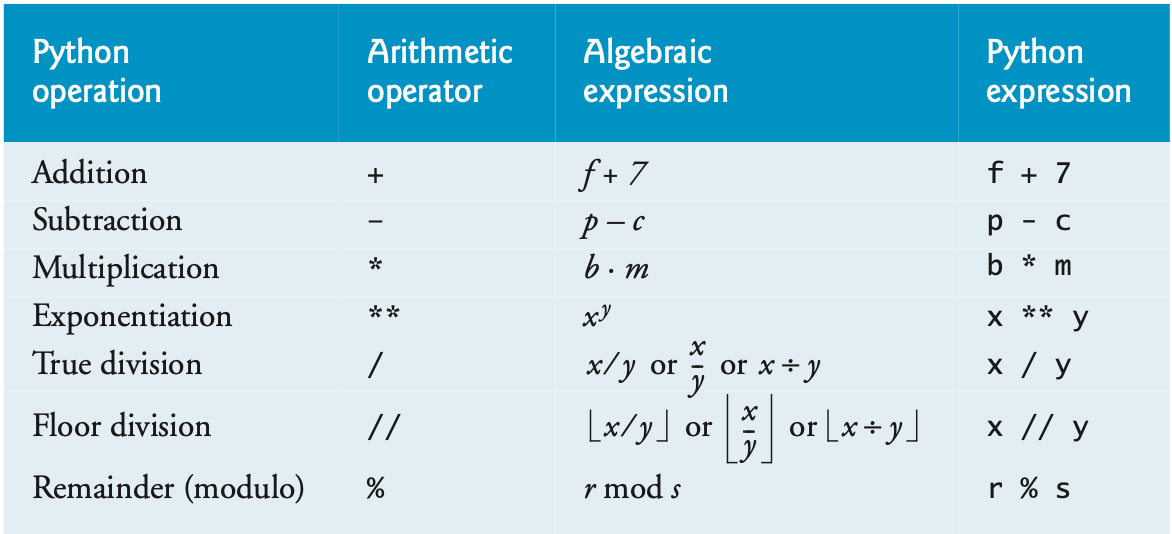
Multiplication:
Rather than algebra’s center dot (·), Python uses the asterisk (*) multiplication operator:7 * 4
Exponentiation:
The exponentiation (**) operator raises one value to the power of another: ```python 2 ** 10 ``` To calculate the square root, you can use the exponent 1/2 (that is, 0.5): ```python 9 ** (1/2) ```True Division (/) vs. Floor Division (//):
True division (/) divides a numerator by a denominator and yields a floating-point number with a decimal point, as in: ```python print(7 / 4) # This will display 1.75 ``` Floor division (//) divides a numerator by a denominator, yielding the highest integer that’s not greater than the result. Python truncates (discards) the fractional part: ```python print(7 // 4) # This will display 1 ``` Dividing by zero with / or // is not allowed and results in an exception —- a sign that a problem occurred:
Python reports an exception with a traceback. This traceback indicates that an exception of type ZeroDivisionError occurred. If it helps, most exception names end with Error.
The line that begins with ----> 1
shows the code that caused the exception. The last line shows the exception that occurred, followed by a colon (:) and an error message with more information about the exception. In this case, the exception that occurred is ZeroDivisionError: division by zero
.
An exception also occurs if you try to use a variable that you have not yet created. The following code segment tries to add 7 to the undefined variable z, resulting in a NameError:

Python’s remainder operator (%
) yields the remainder after the left operand is divided by the right operand:
print(17 % 5) # This will display 2
In this case, 17 divided by 5 yields a quotient of 3 and a remainder of 2. This operator is most commonly used with integers, but also can be used with other numeric types.
Operator Precedence Rules:
Python applies the operators in arithmetic expressions according to the following rules of operator precedence. These are generally the same as those in algebra:
Expressions in parentheses evaluate first, so parentheses may force the order of evaluation to occur in any sequence you desire. Parentheses have the highest level of precedence. In expressions with nested parentheses, such as (a / (b - c)), the expression in the innermost parentheses (that is, b - c) evaluates first.
Exponentiation operations evaluate next. If an expression contains several exponentiation operations, Python applies them from right to left.
Multiplication, division and modulus operations evaluate next. If an expression contains several multiplication, true-division, floor-division and modulus operations, Python applies them from left to right. Multiplication, division and modulus are “on the same level of precedence.”
Addition and subtraction operations evaluate last. If an expression contains several addition and subtraction operations, Python applies them from left to right. Addition and subtraction also have the same level of precedence.
Operator Grouping:
When we say that Python applies certain operators from left to right, we are referring to the operators’ grouping. For example, in the expression `a+b+c`, the addition operators (+) group from left to right as if we parenthesized the expression as (a + b) + c. All Python operators of the same precedence group left-to-right except for the exponentiation operator (**), which groups right-to-left.Redundant Parentheses:
You can use redundant parentheses to group subexpressions to make the expression clearer. For example, the second-degree polynomialy = a * x ** 2 + b * x + c
can be parenthesized, for clarity, as
y = (a * (x ** 2)) + (b * x) + c
Breaking a complex expression into a sequence of statements with shorter, simpler expressions also can promote clarity.