Strings¶
The print
statement displays some value as a line of text:
print('Welcome to Python!') # This displays the sentence "Welcome to Python!"
In this case, the sentence ‘Welcome to Python!’ is a string -— a sequence of characters enclosed in single quotes (‘).
You also may enclose a string in double quotes (“), as in:
print("Welcome to Python!") # This displays the sentence "Welcome to Python!"
Python programmers generally prefer single quotes, but choose whichever style feels best for you!
When print completes its task, it positions the screen cursor at the beginning of the next line. This is similar to what happens when you press the Enter (or Return) key while typing in a text editor.
The print function can receive a comma-separated list of arguments, as in:
print('Welcome', 'to', 'Python!') # This displays the sentence "Welcome to Python!"
The print statement displays each string separated from the next by a space, producing the same output as in the two preceding code segments. Here we showed a comma-separated list of strings, but the values can be of any type.
Escape Characters
When a backslash (\) appears in a string, it’s known as the escape character. The backslash and the character immediately following it form an escape sequence. For example, \n represents the newline character escape sequence, which tells print to move the output cursor to the next line. Placing two newline characters back-to-back displays a blank line. The following snippet uses three newline characters to create many lines of output:print('Welcome\nto\n\nPython!')
Other Escape Sequences:
The following table shows some common escape sequences.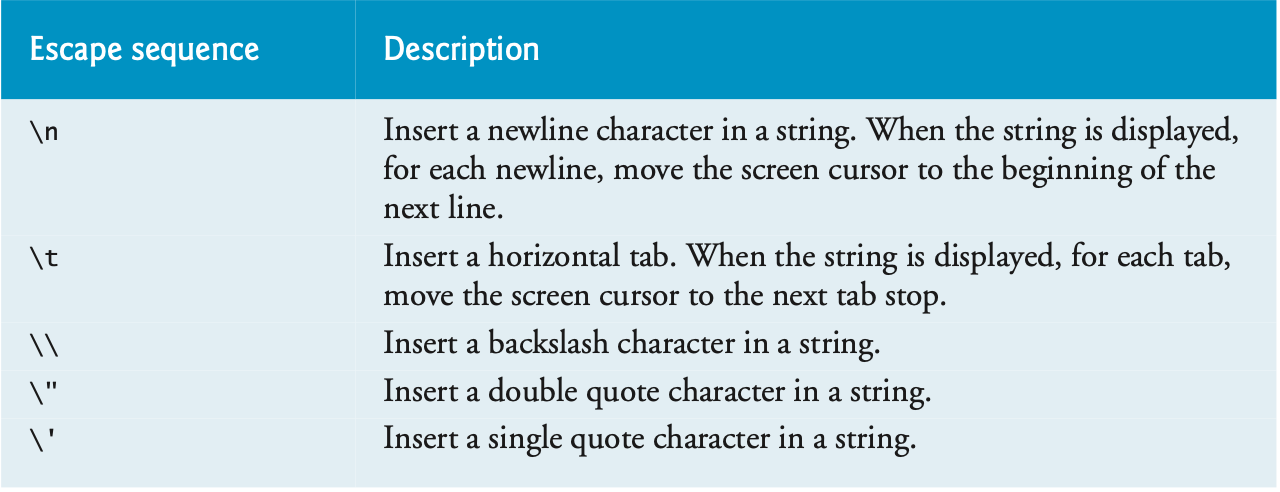
Triple-quoted Strings:
Triple-quoted strings begin and end with three double quotes (""") or three single quotes ('''). The Style Guide for Python Code recommends three double quotes ("""). Use these to create multiline strings and strings containing single or double quotes.Including Quotes in Strings:
In a string delimited by single quotes, you may include double-quote characters: ```Python print('Display "hi" in quotes') ``` but not single quotes:print('Display `hi` in quotes')
The second code segment will display a syntax error, which is a violation of Python’s language rules. In this case, a single quote inside a single-quoted string is a no-no! You will most likely see something that looks like this:

This message displays information about the line of code that caused the syntax error and points to the error with a ^
symbol. It also displays the message SyntaxError: invalid syntax
.
Multi-line Strings:
Triple quotes can be used to write a long string over multiple lines:
print("""Line 1 is a long line. I need more space!
I am continuing to write, so I need another line""")