Boolean Operators¶
The conditional operators >, <, >=, <=, == and != can be used to form simple conditions such as grade >= 60. To form more complex conditions that combine simple conditions, use the and, or and not Boolean operators.
Boolean Operator and:
To ensure that two conditions are both True before executing a control statement’s suite, use the Boolean `and` operator to combine the conditions. The following code defines two variables, then tests a condition that’s True if and only if both simple conditions are True— if either (or both) of the simple conditions is False, the entire and expression is False:gender = 'Female'
age = 70
if gender == 'Female' and age >= 60:
print('Senior female')
The if statement has two simple conditions:
gender == 'Female'
determines whether a person is a female andage >= 65
determines whether that person is a senior citizen.
The simple condition to the left of the and
operator evaluates first because == has higher precedence than and
. If necessary, the simple condition to the right of and
evaluates next, because >=
has higher precedence than and
. The entire if statement condition is True if and only if both of the simple conditions are True. The combined condition can be made clearer by adding redundant (unnecessary) parentheses: (gender == 'Female') and (age >= 65)
.
The table below summarizes the and
operator by showing all four possible combinations of False and True values for expression1 and expression2—such tables are called truth tables:
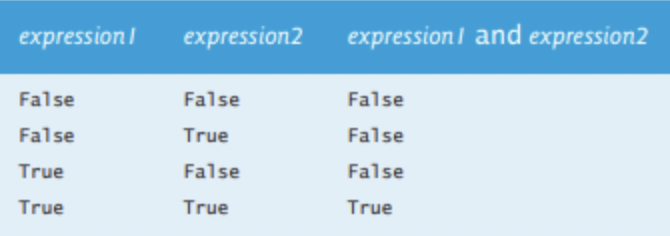
Boolean Operator or:
Use the Boolean `or` operator to test whether one or both of two conditions are True. The following code tests a condition that’s True if either or both simple conditions are True— the entire condition is False only if both simple conditions are False:semester_average = 83
final_exam = 95
if semester_average >= or final_exam >= 90:
print('Student gets an A')
The code segment above also contains two simple conditions:
semester_average >= 90
determines whether a student’s average was an A (90 or above) during the semester, andfinal_exam >= 90
determines whether a student’s final-exam grade was an A.
The truth table below summarizes the Boolean or
operator. Operator and has higher precedence than or.
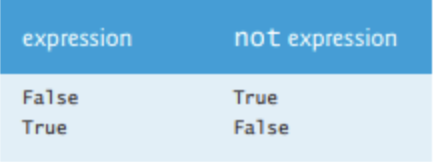
Boolean Operator not
The Boolean `not` operator “reverses” the meaning of a condition—True becomes False and False becomes True. This is a unary operator—it has only one operand. You place the not operator before a condition to choose a path of execution if the original condition (without the not operator) is False, such as in the following code:grade = 59
if not grade == -1:
print('The next grade is…', grade)
Often, you can avoid using not
by expressing the condition in a more “natural” or convenient manner. For example, the preceding if statement can also be written as follows:
grade = 59
if grade != -1:
print('The next grade is…', grade)
The truth table below summarizes the not operator.
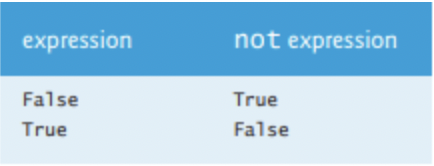
The following table shows the precedence and grouping of the operators introduced so far, from top to bottom, in decreasing order of precedence.
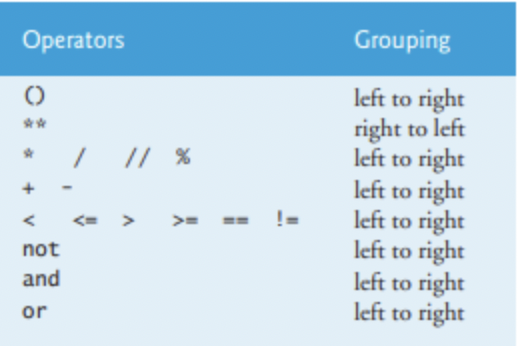