If statements¶
We now present a simple version of the if statement, which uses a condition to decide whether to execute a statement (or a group of statements). Here we’ll ask a user to input two integers and compare them using six consecutive if statements, one for each comparison operator. If the condition in a given if statement is True, the corresponding print statement executes; otherwise, it’s skipped.
print('Enter two integers, and I will tell you the',
' relationship they satisfy.')
# get first integer
num1 = int(input('Enter first integer: '))
# get second integer
num2 = int(input('Enter second integer: '))
if num1 == num2:
print(num1, 'is equal to', num2)
if num1 == num2:
print(num1, 'is not equal to', num2)
if num1 < num2:
print(num1, 'is less than', num2)
if num1 > num2:
print(num1, 'is greater than', num2)
This is what it might look like to run this program:
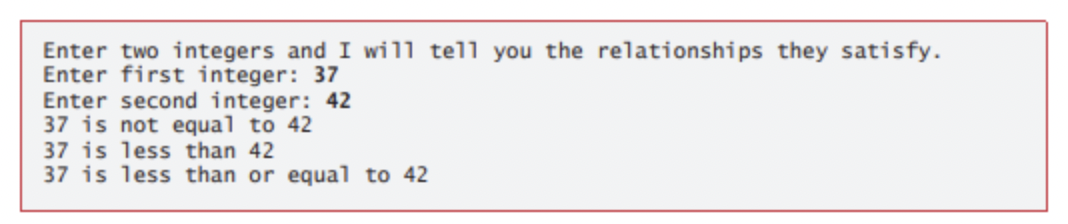
Pay attention to which conditions become True. This will trigger the print statement of these if statements to run. For example, the if statement found on lines 13–14 looks like this: if number1 == number2: print(number1, 'is equal to', number2)
. More specifically, the condition uses the == comparison operator to determine whether the values of variables number1 and number2 are equal. If so, the condition is True, and line 14 displays a line of text indicating that the values are equal. If any of the remaining if statements’ conditions are True, the corresponding print displays a line of text. Each if statement consists of the keyword if
, the condition to test, and a colon (:) followed by an indented body of statements. Each indented body of an if statement must contain one or more statements. Forgetting the colon (:) after the condition is a common syntax error.
Indentation:
Python requires you to ***indent*** the statements found in the body of an if statement. Python will automatically indent the line of code found after a color (:) but just in case it doesn’t happen, press the tab key on your keyboard. Incorrect indentation can cause errors! So be careful when you are writing if statements in your programs.Confusing == and =
Using the assignment symbol (=) instead of the equality operator (==) in an if statement’s condition is a common mistake. To help avoid this, read == as “is equal to” and = as “is assigned.”Chaining Comparisons
You can chain comparisons to check whether a value is in a range. The following comparison determines whether x is in the range 1 through 5, inclusive:x = 3
1<= x <=5